Create a Connection
Create a connection to give a producer access to quote a product.
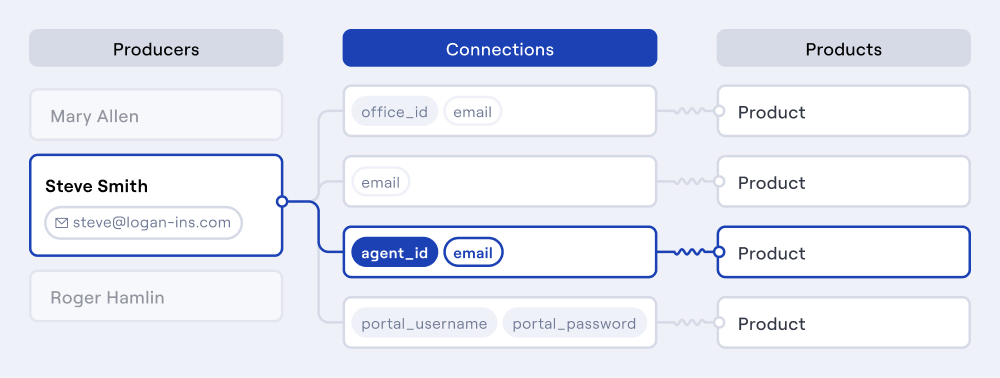
Creating a connection gives a producer the access to get quotes for a product via API. A connection is the relationship between an individual producer and an individual product, requiring any relevant [.h-code-link]auth_values[.h-code-link] to authenticate the identity and appointment of the producer.
The relevant [.h-code]auth_values[.h-code] to connect a producer to a given product are documented in the Producer Connection Appendix, or can be retrieved dynamically by creating a Connections Intent. Only some products require authentication values, since some products authenticate a producer using information stored in the producer object like the producer’s email address.
Each connection is given a [.h-code]status[.h-code] of either active or referred. If the connection has an [.h-code]active[.h-code] status, the producer is authenticated and able to get quotes for the product. A [.h-code]referred[.h-code] connection requires more information from the carrier or Herald's team, and should be resolved in less than 24 hours. If a producer attempts to submit an application to quote a product they are not connected to, or with a [.h-code]referred[.h-code] status, it will result in a [.h-code]400[.h-code].
Create a Connection
To make a connection, use [.h-code]POST[.h-code] [.h-endpoint-link]/connections[.h-endpoint-link] including the [.h-code]producer_id[.h-code] and [.h-code]product_id[.h-code] you want to connect. If the connection requires authentication values, include each value in the [.h-code-link]auth_values[.h-code-link] array. If authentication values are not required, you can either submit an empty array or remove [.h-code-link]auth_values[.h-code-link] all together.
Below are example requests for a connection that requires authentication values, and a connection that does not.
In both examples, the response includes the details you’ve submitted as well as an [.h-code]id[.h-code] and a [.h-code]status[.h-code].
- The [.h-code]id[.h-code] can be used to get the details of the connection or update the connection.
- The [.h-code]status[.h-code] of a connection communicates if the producer is able to get quotes for the product.
[.icon-circle-blue][.icon-circle-blue] Note that when a connection requires authentication values, all values must be submitted in order to make the connection.
Connection Status
Each connection you create is given a [.h-code]status[.h-code] of active or referred.
- A connection with an [.h-code]active[.h-code] status communicates that the producer is connected to the product and ready to make submissions.
- A connection with a [.h-code]referred[.h-code] status means a review is needed, and this was sent to Herald's team to complete the connection. It’s important to note, a referred status does not mean more information is needed from you.
In many cases, a connection will have an [.h-code]active[.h-code] status immediately after you’ve created it. This means you can create a producer, connect them to a product, and give them the ability to submit an application for a quote in a matter of seconds.
Creating a connection that results in a referred status is most likely when you connect to a product for the first time. As explained in our connections doc, a carrier may require their own form of authentication to confirm the identity and appointment of a producer, and Herald collects these representations as [.h-code-link]auth_values[.h-code-link]. Common examples of these forms of authentication include a producers username and password to log into a carriers portal.
In some cases, a carrier may require representation of entities above the producer, such as an [.h-code]api_key[.h-code] for your platform to access their API. These values may represent your platform or an individual distributor, and are typically only known by the carrier rather than a value you are familiar with. In these cases Herald submits those values behind-the-scenes when you integrate with a product for the first time, and they are reused for all subsequent connections you make in the future.
[.icon-circle-blue][.icon-circle-blue] If you receive a [.h-code]referred[.h-code] status, assume that Herald has been notified and is taking care of it! Use [.h-code]GET[.h-code] [.h-endpoint-link]/connections/{connection_id}[.h-endpoint-link] to see if a connection status has been updated from [.h-code]referred[.h-code] to [.h-code]active[.h-code].
Get a Producer's Connections
Once you’ve created a connection, the details of the connection will appear in the [.h-code]connections[.h-code] array of each producer object. You can see an individual producer's connections using [.h-code]GET[.h-code] [.h-endpoint-link]/producers/{producer_id}[.h-endpoint-link]. Read more in our guide to managing producers.
Following the example above, the newly created connection would appear in the producer object like this:
Update a Connection
You can update a connection using [.h-code]PUT[.h-code] [.h-endpoint-link]/connections/{connection_id}[.h-endpoint-link], which allows you to update the [.h-code]value[.h-code] of an [.h-code]auth_value[.h-code]. You cannot update the [.h-code]producer_id[.h-code] or [.h-code]product_id[.h-code] for a connection, create a new connection instead.
This endpoint is useful if a producer updates information such as their username or password for a carrier's portal. Keeping this information up to date is the best way to prevent errors when making submissions.
[.icon-circle-blue][.icon-circle-blue] When you submit an application, [.h-code]auth_values[.h-code] are used to authenticate with the carriers API. Updating a value with inaccurate information may result in an error when making a submission.
Let’s look at an example where we need to update the username and password for this connection.
Get a Connection
You can get the details of an individual connection using [.h-code]GET[.h-code] [.h-endpoint-link]/connections/{connection_id}[.h-endpoint-link]. Getting the details of a connection is useful when checking for an updated [.h-code]status[.h-code] of a connection.